타입스크립트 완벽 가이드: 기초부터 고급 기능까지
타입스크립트는 현대 웹 개발에서 필수적인 도구로 자리 잡았습니다. 자바스크립트의 모든 기능을 포함하면서도 정적 타입 시스템을 통해 더 안전하고 유지보수하기 쉬운 코드를 작성할 수 있게 해줍니다. 이 글에서는 타입스크립트의 기초부터 고급 기능까지 상세히 알아보겠습니다.
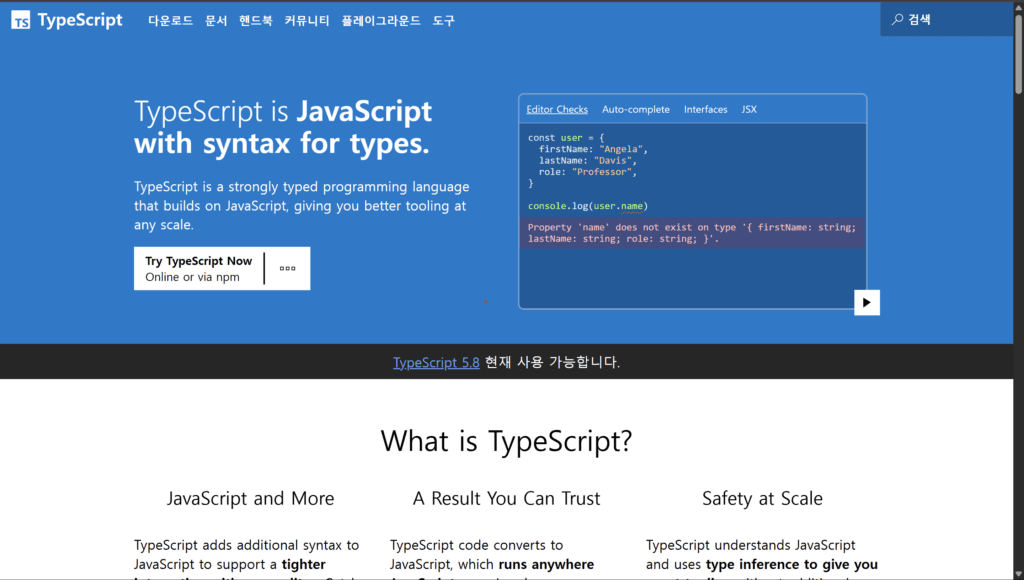
타입스크립트란 무엇인가?
타입스크립트는 마이크로소프트에서 개발한 오픈 소스 프로그래밍 언어로, 자바스크립트의 상위 집합(Superset)입니다. 자바스크립트의 모든 기능을 포함하면서도 정적 타입 시스템을 추가하여 코드의 안정성과 가독성을 높여줍니다.
타입스크립트의 핵심 특징
타입스크립트는 “점진적 타입 시스템”을 채택하여 정적 타입 시스템과 동적 타입 시스템의 장점을 모두 활용합니다:
- 정적 타입 시스템처럼 코드 실행 전 타입 검사 수행
- 동적 타입 시스템처럼 모든 변수에 타입을 명시할 필요 없음
- 타입 추론을 통해 초기값을 기준으로 자동으로 타입 결정
타입스크립트의 주요 이점
- 버그 감소: 컴파일 시점에 타입 오류를 발견하여 런타임 오류 감소
- 코드 가독성 향상: 타입 정보가 문서 역할을 하여 코드 이해도 증가
- 개발 생산성 향상: 코드 자동 완성, 리팩토링 등 IDE 지원 강화
- 안전한 리팩토링: 타입 시스템이 코드 변경 시 발생할 수 있는 문제 사전 감지
타입스크립트 설치 및 환경 설정
타입스크립트를 시작하기 위해서는 Node.js와 npm이 필요합니다.
전역 설치
bashnpm install -g typescript
프로젝트별 설치
bashnpm init -y
npm install typescript --save-dev
tsconfig.json 설정
프로젝트 루트에 tsconfig.json 파일을 생성하여 타입스크립트 컴파일러 옵션을 설정할 수 있습니다:
json{
"compilerOptions": {
"target": "es6",
"module": "commonjs",
"outDir": "./dist",
"rootDir": "./src",
"strict": true,
"esModuleInterop": true
},
"include": ["src/**/*"],
"exclude": ["node_modules"]
}
타입스크립트 기본 타입
타입스크립트는 다양한 기본 타입을 제공합니다.
기본 타입
typescript// 기본 타입
let isDone: boolean = false;
let decimal: number = 6;
let color: string = "blue";
let list: number[] = [1, 2, 3];
let tuple: [string, number] = ["hello", 10];
특수 타입
typescript// any 타입 - 모든 타입 허용
let notSure: any = 4;
notSure = "maybe a string";
// unknown 타입 - any보다 안전한 타입
let value: unknown = 10;
if (typeof value === "number") {
let num: number = value; // 타입 체크 후 할당 가능
}
// void 타입 - 반환값이 없는 함수
function warnUser(): void {
console.log("Warning message");
}
// null과 undefined
let u: undefined = undefined;
let n: null = null;
객체 타입
typescript// 객체 타입 정의
let user: { name: string; age: number } = {
name: "John",
age: 30
};
// 인터페이스를 사용한 객체 타입 정의
interface User {
name: string;
age: number;
email?: string; // 선택적 속성
}
let newUser: User = {
name: "Jane",
age: 25
};
인터페이스와 타입 별칭
타입스크립트에서는 인터페이스와 타입 별칭을 통해 복잡한 타입을 정의할 수 있습니다.
인터페이스(Interface)
인터페이스는 객체의 구조를 정의하는 방법입니다.
typescriptinterface Person {
firstName: string;
lastName: string;
age?: number; // 선택적 속성
readonly id: number; // 읽기 전용 속성
sayHello(): string; // 메서드 시그니처
}
const john: Person = {
firstName: "John",
lastName: "Doe",
id: 1,
sayHello() {
return `Hello, I'm ${this.firstName}`;
}
};
인터페이스 확장
인터페이스는 다른 인터페이스를 확장할 수 있습니다.
typescriptinterface Employee extends Person {
jobTitle: string;
salary: number;
}
const jane: Employee = {
firstName: "Jane",
lastName: "Smith",
id: 2,
jobTitle: "Developer",
salary: 50000,
sayHello() {
return `Hello, I'm ${this.firstName}, a ${this.jobTitle}`;
}
};
타입 별칭(Type Alias)
타입 별칭은 인터페이스와 유사하지만 더 다양한 타입을 정의할 수 있습니다.
typescripttype Point = {
x: number;
y: number;
};
// 유니온 타입
type ID = string | number;
// 튜플 타입
type Coordinates = [number, number];
// 함수 타입
type CalculateFunction = (a: number, b: number) => number;
인터페이스 vs 타입 별칭
두 방식 모두 타입을 정의하는 데 사용되지만 몇 가지 차이점이 있습니다:
- 인터페이스는 선언 병합(declaration merging)이 가능하지만 타입 별칭은 불가능
- 타입 별칭은 유니온, 교차 타입 등 더 다양한 타입 표현이 가능
- 인터페이스는 객체, 클래스의 구조를 정의하는 데 더 적합
함수 타입
타입스크립트에서는 함수의 매개변수와 반환 타입을 명시할 수 있습니다.
함수 타입 정의
typescript// 함수 선언식
function add(a: number, b: number): number {
return a + b;
}
// 함수 표현식
const subtract = function(a: number, b: number): number {
return a - b;
};
// 화살표 함수
const multiply = (a: number, b: number): number => a * b;
선택적 매개변수와 기본값
typescript// 선택적 매개변수
function greet(name: string, greeting?: string): string {
return greeting ? `${greeting}, ${name}!` : `Hello, ${name}!`;
}
// 기본값 매개변수
function greetWithDefault(name: string, greeting: string = "Hello"): string {
return `${greeting}, ${name}!`;
}
나머지 매개변수
typescriptfunction sum(...numbers: number[]): number {
return numbers.reduce((total, num) => total + num, 0);
}
console.log(sum(1, 2, 3, 4)); // 10
함수 오버로드
typescript// 함수 오버로드 시그니처
function process(value: string): string;
function process(value: number): number;
function process(value: string | number): string | number {
if (typeof value === "string") {
return value.toUpperCase();
} else {
return value * 2;
}
}
console.log(process("hello")); // "HELLO"
console.log(process(10)); // 20
클래스와 상속
타입스크립트는 객체지향 프로그래밍을 위한 클래스와 상속을 지원합니다.
기본 클래스 정의
typescriptclass Animal {
name: string;
constructor(name: string) {
this.name = name;
}
makeSound(): void {
console.log("Some generic sound");
}
}
const animal = new Animal("Generic Animal");
animal.makeSound(); // "Some generic sound"
접근 제한자
typescriptclass Person {
public name: string; // 어디서나 접근 가능
private age: number; // 클래스 내부에서만 접근 가능
protected email: string; // 클래스와 상속받은 클래스에서 접근 가능
readonly id: number; // 읽기 전용 속성
constructor(name: string, age: number, email: string, id: number) {
this.name = name;
this.age = age;
this.email = email;
this.id = id;
}
public getAge(): number {
return this.age;
}
}
상속
typescriptclass Dog extends Animal {
breed: string;
constructor(name: string, breed: string) {
super(name); // 부모 클래스의 생성자 호출
this.breed = breed;
}
makeSound(): void {
console.log("Woof! Woof!");
}
getInfo(): string {
return `${this.name} is a ${this.breed}`;
}
}
const dog = new Dog("Rex", "German Shepherd");
dog.makeSound(); // "Woof! Woof!"
console.log(dog.getInfo()); // "Rex is a German Shepherd"
추상 클래스
typescriptabstract class Shape {
color: string;
constructor(color: string) {
this.color = color;
}
abstract calculateArea(): number; // 추상 메서드
displayColor(): void {
console.log(`This shape is ${this.color}`);
}
}
class Circle extends Shape {
radius: number;
constructor(color: string, radius: number) {
super(color);
this.radius = radius;
}
calculateArea(): number {
return Math.PI * this.radius * this.radius;
}
}
const circle = new Circle("red", 5);
console.log(circle.calculateArea()); // 78.54...
circle.displayColor(); // "This shape is red"
제네릭(Generics)
제네릭은 타입을 매개변수로 사용하여 재사용 가능한 컴포넌트를 만들 수 있게 해줍니다.
제네릭 함수
typescriptfunction identity<T>(arg: T): T {
return arg;
}
// 명시적 타입 지정
let output1 = identity<string>("myString");
// 타입 추론
let output2 = identity(42); // 타입은 number로 추론됨
제네릭 인터페이스
typescriptinterface Box<T> {
value: T;
}
let stringBox: Box<string> = { value: "hello" };
let numberBox: Box<number> = { value: 42 };
제네릭 클래스
typescriptclass Queue<T> {
private data: T[] = [];
push(item: T): void {
this.data.push(item);
}
pop(): T | undefined {
return this.data.shift();
}
}
const numberQueue = new Queue<number>();
numberQueue.push(10);
numberQueue.push(20);
console.log(numberQueue.pop()); // 10
const stringQueue = new Queue<string>();
stringQueue.push("hello");
stringQueue.push("world");
console.log(stringQueue.pop()); // "hello"
제네릭 제약조건
typescriptinterface Lengthwise {
length: number;
}
function loggingIdentity<T extends Lengthwise>(arg: T): T {
console.log(arg.length); // .length 속성이 있음을 보장
return arg;
}
loggingIdentity("hello"); // 5
loggingIdentity([1, 2, 3]); // 3
// loggingIdentity(3); // 오류: number에는 .length 속성이 없음
고급 타입
타입스크립트는 더 복잡한 타입 관계를 표현할 수 있는 고급 타입 기능을 제공합니다.
유니온 타입(Union Types)
typescript// 여러 타입 중 하나를 가질 수 있음
function printId(id: number | string) {
if (typeof id === "string") {
console.log(id.toUpperCase());
} else {
console.log(id);
}
}
printId(101); // 101
printId("202"); // "202"
교차 타입(Intersection Types)
typescript// 여러 타입을 결합
type Employee = {
id: number;
name: string;
};
type Manager = {
department: string;
level: number;
};
type ManagerEmployee = Employee & Manager;
const manager: ManagerEmployee = {
id: 1,
name: "John",
department: "IT",
level: 2
};
타입 가드(Type Guards)
typescript// typeof 타입 가드
function padLeft(value: string, padding: string | number) {
if (typeof padding === "number") {
return " ".repeat(padding) + value;
}
return padding + value;
}
// instanceof 타입 가드
class Bird {
fly() {
console.log("flying...");
}
}
class Fish {
swim() {
console.log("swimming...");
}
}
function move(animal: Bird | Fish) {
if (animal instanceof Bird) {
animal.fly();
} else {
animal.swim();
}
}
리터럴 타입(Literal Types)
typescript// 특정 값만 허용하는 타입
type Direction = "north" | "east" | "south" | "west";
function move(direction: Direction) {
console.log(`Moving ${direction}`);
}
move("north"); // OK
// move("northeast"); // 오류: "northeast"는 Direction 타입이 아님
타입 단언(Type Assertions)
typescript// 타입 단언
let someValue: unknown = "this is a string";
let strLength: number = (someValue as string).length;
// 또는 각괄호 구문 (JSX에서는 사용 불가)
let strLength2: number = (<string>someValue).length;
모듈과 네임스페이스
타입스크립트는 코드를 구조화하기 위한 모듈과 네임스페이스 시스템을 제공합니다.
ES 모듈
typescript// math.ts
export function add(x: number, y: number): number {
return x + y;
}
export function subtract(x: number, y: number): number {
return x - y;
}
// app.ts
import { add, subtract } from './math';
console.log(add(5, 3)); // 8
console.log(subtract(5, 3)); // 2
기본 내보내기와 가져오기
typescript// person.ts
export default class Person {
constructor(public name: string) {}
greet() {
return `Hello, my name is ${this.name}`;
}
}
// app.ts
import Person from './person';
const person = new Person("John");
console.log(person.greet()); // "Hello, my name is John"
네임스페이스
typescript// validation.ts
namespace Validation {
export interface StringValidator {
isValid(s: string): boolean;
}
export class EmailValidator implements StringValidator {
isValid(s: string): boolean {
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return emailRegex.test(s);
}
}
}
// app.ts
/// <reference path="validation.ts" />
let emailValidator = new Validation.EmailValidator();
console.log(emailValidator.isValid("[email protected]")); // true
데코레이터
데코레이터는 클래스, 메서드, 속성 등에 메타데이터를 추가하거나 동작을 변경할 수 있는 기능입니다. 현재 JavaScript에서는 Stage 3 단계에 있으며, TypeScript에서는 이미 구현되어 있습니다8.
클래스 데코레이터
typescriptfunction sealed(constructor: Function) {
Object.seal(constructor);
Object.seal(constructor.prototype);
}
@sealed
class BugReport {
type = "report";
title: string;
constructor(title: string) {
this.title = title;
}
}
메서드 데코레이터
typescriptfunction log(target: any, propertyKey: string, descriptor: PropertyDescriptor) {
const originalMethod = descriptor.value;
descriptor.value = function(...args: any[]) {
console.log(`Calling ${propertyKey} with arguments: ${JSON.stringify(args)}`);
const result = originalMethod.apply(this, args);
console.log(`Method ${propertyKey} returned: ${JSON.stringify(result)}`);
return result;
};
return descriptor;
}
class Calculator {
@log
add(a: number, b: number): number {
return a + b;
}
}
const calc = new Calculator();
calc.add(1, 2);
// "Calling add with arguments:"
// "Method add returned: 3"
실행 시간 측정 데코레이터
typescriptfunction measure(target: Function, context) {
if (context.kind === 'method') {
return function(...args: any[]) {
const start = performance.now();
const result = target.apply(this, args);
const end = performance.now();
console.log(`Execution time: ${end - start} milliseconds`);
return result;
};
}
}
class Rocket {
fuel = 100;
@measure
launch() {
console.log("Launching in 3... 2... 1... 🚀");
return true;
}
}
const rocket = new Rocket();
rocket.launch();
// "Launching in 3... 2... 1... 🚀"
// "Execution time: 1.062355000525713 milliseconds"
데코레이터 팩토리와 조합
데코레이터 팩토리는 데코레이터에 매개변수를 전달할 수 있게 해주는 함수입니다8:
typescriptfunction minimumFuel(fuel: number) {
return function(target: Function, context) {
if (context.kind === 'method') {
return function(...args: any[]) {
if (this.fuel > fuel) {
console.log('fuel is more than mininum');
return target.apply(this, args);
} else {
console.log(`Not enough fuel. Required: ${fuel}, got ${this.fuel}`);
}
};
}
};
}
function maximumFuel(fuel: number) {
return function(target: Function, context) {
if (context.kind === 'method') {
return function(...args: any[]) {
if (this.fuel < fuel) {
console.log('fuel is less than maximum');
return target.apply(this, args);
} else {
console.log(`excessive fuel. Maximum: ${fuel}, got ${this.fuel}`);
}
};
}
};
}
타입스크립트 컴파일 과정
타입스크립트 코드가 자바스크립트로 변환되는 과정을 이해하는 것은 중요합니다.
컴파일 단계
- 타입스크립트 코드 작성
- .ts 또는 .tsx 확장자 파일에 코드를 작성합니다9.
- AST(추상 구문 트리) 변환
- 타입스크립트 컴파일러가 코드를 AST로 변환합니다.
- 타입 검사
- AST를 기반으로 코드의 타입 오류를 검사합니다.
- 자바스크립트 코드 생성
- 타입 검사를 통과한 AST를 자바스크립트 코드로 변환합니다.
타입스크립트 컴파일러 설치
타입스크립트 컴파일러(tsc)는 npm을 통해 설치할 수 있습니다9:
bashnpm install -g typescript
또는 프로젝트 로컬에 설치:
bashnpm install --save-dev typescript
tsconfig.json 주요 옵션
json{
"compilerOptions": {
"target": "es2020", // 출력 JS 버전
"module": "commonjs", // 모듈 시스템
"strict": true, // 엄격한 타입 검사
"esModuleInterop": true, // 모듈 상호 운용성
"outDir": "./dist", // 출력 디렉토리
"rootDir": "./src", // 소스 디렉토리
"declaration": true, // .d.ts 파일 생성
"sourceMap": true, // 소스맵 생성
"noImplicitAny": true, // 암시적 any 금지
"strictNullChecks": true // null, undefined 엄격 검사
}
}
간단한 컴파일 예제
- 타입스크립트 파일 생성 (helloworld.ts)9:
typescriptlet message: string = 'Hello World';
console.log(message);
- 컴파일 명령어 실행:
bashtsc helloworld.ts
- 생성된 자바스크립트 파일 실행:
bashnode helloworld.js
타입스크립트와 리액트
타입스크립트는 리액트와 함께 사용할 때 특히 강력합니다3.
리액트 컴포넌트 타입 정의
typescriptimport React, { FC } from 'react';
interface UserProps {
name: string;
age: number;
isAdmin?: boolean; // 선택적 prop
}
const User: FC<UserProps> = ({ name, age, isAdmin = false }) => {
return (
<div>
<h1>{name}</h1>
<p>Age: {age}</p>
{isAdmin && <p>Admin User</p>}
</div>
);
};
// 사용 예
const App: FC = () => {
return (
<div>
<User name="John" age={30} isAdmin={true} />
<User name="Jane" age={25} />
</div>
);
};
상태 관리와 이벤트 핸들링
typescriptimport React, { useState, ChangeEvent, FormEvent } from 'react';
interface FormState {
email: string;
password: string;
}
const LoginForm: React.FC = () => {
const [formData, setFormData] = useState<FormState>({
email: '',
password: ''
});
const handleChange = (e: ChangeEvent<HTMLInputElement>) => {
const { name, value } = e.target;
setFormData(prev => ({
...prev,
[name]: value
}));
};
const handleSubmit = (e: FormEvent<HTMLFormElement>) => {
e.preventDefault();
console.log('Form submitted:', formData);
};
return (
<form onSubmit={handleSubmit}>
<div>
<label htmlFor="email">Email:</label>
<input
type="email"
id="email"
name="email"
value={formData.email}
onChange={handleChange}
required
/>
</div>
<div>
<label htmlFor="password">Password:</label>
<input
type="password"
id="password"
name="password"
value={formData.password}
onChange={handleChange}
required
/>
</div>
<button type="submit">Login</button>
</form>
);
};
리액트와 타입스크립트 파일 확장자
리액트와 타입스크립트를 함께 사용할 때는 파일 확장자를 주의해야 합니다3:
- JSX를 포함하는 파일:
.tsx
확장자 사용 - JSX를 포함하지 않는 파일:
.ts
확장자 사용
타입스크립트 모범 사례
타입스크립트를 효과적으로 사용하기 위한 모범 사례를 알아보겠습니다4.
타입 안전성 확보
any
타입 사용 최소화하기unknown
타입을 사용하여 타입 안전성 높이기strictNullChecks
활성화하기- 타입 단언보다 타입 가드 사용하기
코드 구조화
- 관련 타입을 함께 그룹화하기
- 재사용 가능한 타입 정의하기
- 네이밍 컨벤션 일관되게 유지하기
- 인터페이스와 타입 별칭 적절히 사용하기
성능 최적화
- 타입 정의 파일(.d.ts) 활용하기
- 불필요한 타입 체크 피하기
- 타입 추론 활용하기
- 번들 크기 최소화하기
ESLint와 Prettier 통합
결론
타입스크립트는 자바스크립트의 모든 기능을 포함하면서도 정적 타입 시스템을 통해 코드의 안정성과 유지보수성을 크게 향상시킵니다67. 기본 타입부터 고급 기능까지 다양한 기능을 제공하여 대규모 애플리케이션 개발에 특히 유용합니다.
타입스크립트를 사용하면 코드 작성 단계에서 오류를 발견하고7, 더 나은 개발자 경험을 제공하며, 코드의 자기 문서화 능력을 높일 수 있습니다. 현대 웹 개발에서 타입스크립트는 선택이 아닌 필수가 되어가고 있으며, 이 가이드가 여러분의 타입스크립트 여정에 도움이 되기를 바랍니다.
자주 묻는 질문 (FAQ)
1. 타입스크립트를 배우는 데 얼마나 시간이 걸리나요?
자바스크립트에 익숙하다면 타입스크립트의 기본 개념을 배우는 데 1-2주 정도 소요됩니다. 하지만 고급 기능과 타입 시스템을 완전히 이해하고 활용하는 데는 몇 개월이 걸릴 수 있습니다.
2. 타입스크립트는 성능에 영향을 미치나요?
타입스크립트는 컴파일 시점에만 타입 검사를 수행하고, 런타임에는 일반 자바스크립트로 변환되어 실행되므로 성능에 직접적인 영향을 미치지 않습니다7. 오히려 타입 시스템을 통해 최적화된 코드를 작성할 수 있습니다.
3. 기존 자바스크립트 프로젝트를 타입스크립트로 마이그레이션하는 방법은 무엇인가요?
점진적으로 마이그레이션하는 것이 좋습니다. 먼저 tsconfig.json 파일을 설정하고, .js 파일을 .ts 파일로 변환하면서 any 타입을 사용하여 시작한 후, 점차 구체적인 타입으로 대체해 나가는 방식을 권장합니다.
4. 타입스크립트와 자바스크립트 라이브러리를 함께 사용할 수 있나요?
네, 대부분의 인기 있는 자바스크립트 라이브러리는 타입 정의 파일(.d.ts)을 제공하거나 DefinitelyTyped(@types/패키지명)를 통해 타입 정의를 설치할 수 있습니다.
5. 타입스크립트의 단점은 무엇인가요?
초기 설정 시간이 필요하고, 학습 곡선이 있으며, 타입 정의로 인해 코드량이 증가할 수 있습니다. 또한 일부 동적 프로그래밍 패턴을 표현하기 어려울 수 있습니다. 하지만 이러한 단점은 타입스크립트가 제공하는 이점에 비하면 크지 않은 경우가 많습니다.
참고 자료
- https://www.reddit.com/r/typescript/comments/ebb3oa/completely_dont_understand_the_purpose_of/
- https://www.semanticscholar.org/paper/7bf1d2fecd8b6871084738069650db7bfcd0d8b1
- https://www.freecodecamp.org/news/use-typescript-with-react/
- https://www.sitepoint.com/react-with-typescript-best-practices/
- https://www.semanticscholar.org/paper/d2908ae12f0ca71253434546e47a4ce1478d4eec
- https://serokell.io/blog/typescript-faqs
- https://www.w3schools.com/typescript/typescript_intro.php
- https://blog.logrocket.com/practical-guide-typescript-decorators/
- https://code.visualstudio.com/docs/typescript/typescript-compiling
- https://www.semanticscholar.org/paper/912970ff9028e24cf8a0a21e49efaa34b439bcc3
- https://www.semanticscholar.org/paper/aa30b3a6f5c774a53e4a53a48d5a6627544a25b2
- https://www.semanticscholar.org/paper/6dceb9cc32de6bcf31089f6f59246847a52158d2
- https://www.semanticscholar.org/paper/d4784249b9aaf0fa7d39b97071277dc0c7d7302b
- https://www.reddit.com/r/typescript/comments/w9viu3/why_use_decorators_at_all/
- https://www.reddit.com/r/typescript/comments/n75mt4/typescript_decorators_in_examples/
- https://www.reddit.com/r/typescript/comments/w772vv/is_anyone_against_using_decorators/
- https://www.reddit.com/r/javascript/comments/yejt7q/askjs_do_you_prefer_to_use_decorators_in_your/
- https://www.reddit.com/r/typescript/comments/1dsice6/typescript_decorators/
- https://www.reddit.com/r/typescript/comments/10qqfl0/decorators_and_dependency_injection/
- https://www.reddit.com/r/typescript/comments/znnmcd/how_often_do_you_use_decorators_and_if_you_do_was/
- https://www.reddit.com/r/typescript/comments/swk7cw/how_to_access_the_current_object_inside_a/
- https://www.reddit.com/r/typescript/comments/m8k0x5/why_doesnt_ts_have_decorators_for_functions/
- https://www.reddit.com/r/typescript/comments/1fkjpfo/are_decorators_really_worth_the_pain/
- https://www.typescriptlang.org/docs/handbook/decorators.html
- https://velog.io/@octo__/TypeScript-%EB%8D%B0%EC%BD%94%EB%A0%88%EC%9D%B4%ED%84%B0Decorator
- https://wiki.yowu.dev/ko/Knowledge-base/TypeScript/Learning/009-decorators-in-typescript-how-to-use-decorators-to-add-functionality-to-your-code
- https://www.typescriptlang.org/ko/docs/handbook/decorators.html
- https://refactoring.guru/ko/design-patterns/decorator/typescript/example
- https://velog.io/@joung5846/TypeScript-Decorator
- https://haeguri.github.io/2019/08/25/typescript-decorator/
- https://velog.io/@kimhalin/TypeScript-Custom-Decorator
- https://partnerjun.tistory.com/61
- https://itmining.tistory.com/88
- https://www.semanticscholar.org/paper/def6dd778acab093187b943f564c0a66b703715f
- https://www.semanticscholar.org/paper/3a6de9bf5e9c2c4eeeb04f8028ef99e9b13b7e7a
- https://www.semanticscholar.org/paper/8a09afba58f6c7766cc3a3eab95e53e0fab5ca15
- https://www.semanticscholar.org/paper/1c9e983d7947d3534edd575c3b046ef81706dc02
- https://www.reddit.com/r/typescript/comments/sfz7xj/recommended_ts_js_build_system/
- https://www.reddit.com/r/typescript/comments/1cm4gjn/compile_typescript_to_js_with_maximum/
- https://www.reddit.com/r/typescript/comments/ckvtj7/where_do_you_stick_your_compiled_javascript_files/
- https://www.reddit.com/r/typescript/comments/1h2gfis/how_do_i_even_run_a_typescript_project/
- https://www.reddit.com/r/webdev/comments/zr1hqa/what_are_the_modern_tools_for_compiling_both/
- https://www.reddit.com/r/node/comments/xkketn/what_tool_do_you_use_to_bundle_your_node/
- https://www.reddit.com/r/typescript/comments/14tyo5y/typescript_compiler_plugin_to_add_js_code/
- https://www.reddit.com/r/typescript/comments/sphnzx/is_it_possible_to_compile_typescript_directly_to/
- https://www.reddit.com/r/typescript/comments/kvm6gs/typescript_compiler_and_obfuscated_code/
- https://www.reddit.com/r/golang/comments/1jblu8m/typescript_compiler_and_go/
- https://www.reddit.com/r/Deno/comments/1gh41ry/why_typescript_if_everything_runs_on_javascript/
- https://www.reddit.com/r/typescript/comments/18bg94c/does_compiled_typescript_incur_a_small/
- https://www.reddit.com/r/typescript/comments/xm3h0u/convert_typescript_to_js_with_jsdoc_annotations/
- https://www.reddit.com/r/typescript/comments/xdil0r/would_it_be_possible_to_create_a_compiler_to/
- https://daily.dev/blog/compile-ts-to-js-a-beginners-guide
- https://www.nextree.io/typescript-compile-process/
- https://stackoverflow.com/questions/12678716/transforming-typescript-into-javascript
- https://www.typescriptlang.org/docs/handbook/compiler-options.html
- https://www.jetbrains.com/help/webstorm/compiling-typescript-to-javascript.html
- https://stackoverflow.com/questions/56117531/at-what-stage-does-typescript-actually-do-compilation
- https://kay0426.tistory.com/39
- https://www.typescriptlang.org/ko/docs/handbook/compiler-options.html
- https://transform.tools/typescript-to-javascript
- https://tedspence.com/improving-on-typescript-package-build-processes-cc0af64849e0
- https://www.semanticscholar.org/paper/7a903d8215e106e9660b61b270f371002d090e41
- https://www.semanticscholar.org/paper/5e66cd1269fd730eb764ba71d60ddab94b558805
- https://www.semanticscholar.org/paper/359be631fb82a6ee70f002b4526a3ef9e8d43c08
- https://pubmed.ncbi.nlm.nih.gov/2408131/
- https://www.reddit.com/r/reactjs/comments/1f7vlxp/best_component_library_for_reactjs_with_typescript/
- https://www.reddit.com/r/reactjs/comments/m0f2xd/how_to_write_a_react_component_in_typescript/
- https://www.reddit.com/r/typescript/comments/m0f392/how_to_write_a_react_component_in_typescript/
- https://www.reddit.com/r/reactjs/comments/1cad3rk/what_do_you_think_about_this_approach_using/
- https://www.reddit.com/r/typescript/comments/lsdt27/nicely_written_complex_react_typescript_project/
- https://www.reddit.com/r/reactjs/comments/1fl5kw5/documenting_the_components_in_the_project_using/
- https://www.reddit.com/r/reactjs/comments/1jhv6tp/best_way_to_learn_react_with_typescript_looking/
- https://www.reddit.com/r/typescript/comments/q4mu2k/open_source_react_project_with_typescript_best/
- https://www.reddit.com/r/reactjs/comments/13lo7xt/document_react_components_with_typescript/
- https://www.reddit.com/r/reactjs/comments/1d7mlg7/typescript_react/
- https://www.reddit.com/r/reactjs/comments/tbn7t8/can_anyone_suggest_any_open_source_react/
- https://www.reddit.com/r/reactjs/comments/hxseh3/naming_convention_for_data_type_vs_component/
- https://www.reddit.com/r/reactjs/comments/1fchfpi/reactjs_in_javascript_or_typescript/
- https://www.reddit.com/r/Frontend/comments/136ee45/great_big_react_typescript_projects/
- https://ddeck.tistory.com/56
- https://github.com/tduyng/react-typescript-example
- https://stackoverflow.com/questions/67968477/creating-a-react-component-with-typescript
- https://react.dev/learn/typescript
- https://github.com/woowa-typescript/woowahan-typescript-with-react-example-code
- https://react-typescript-cheatsheet.netlify.app/docs/basic/getting-started/class_components
- https://tech.cloudmt.co.kr/2023/05/11/reactjs_typescript/
- https://react-typescript-cheatsheet.netlify.app/docs/basic/examples/
- https://velog.io/@velopert/create-typescript-react-component
- https://www.typescriptlang.org/ko/docs/handbook/react.html
- https://codesandbox.io/examples/package/typescript-react
- https://woongtech.tistory.com/entry/%EB%A6%AC%EC%95%A1%ED%8A%B8-%EC%BB%B4%ED%8F%AC%EB%84%8C%ED%8A%B8-%EC%9D%B4%ED%95%B4%ED%95%98%EA%B8%B0-reactcomponenttypescript
- https://www.typescriptlang.org/docs/handbook/react.html
- https://kentcdodds.com/blog/how-to-write-a-react-component-in-typescript
- https://www.semanticscholar.org/paper/7c27c0dd8544b8c6c5dfc406bd3b8ab33508baac
- https://www.semanticscholar.org/paper/54ebd6e9cd3792377ebc546d309777113ee919e8
- https://www.semanticscholar.org/paper/26d49124fc1c79dfde096e8393fbf0ddb0671403
- https://www.ncbi.nlm.nih.gov/pmc/articles/PMC11466809/
- https://www.reddit.com/r/typescript/comments/jlhuc4/good_practices_to_follow_for_a_typescript_project/
- https://www.reddit.com/r/typescript/comments/bmpjwl/can_i_use_airbnb_code_style_guide_with_typescript/
- https://www.reddit.com/r/Frontend/comments/15dyb3j/15_advanced_typescript_tips_for_development/
- https://www.reddit.com/r/typescript/comments/13lw48z/what_best_practices_you_should_learn_before_you/
- https://www.reddit.com/r/typescript/comments/1510frm/any_good_guide_to_know_how_to_write_typescript/
- https://www.reddit.com/r/typescript/comments/1640uml/what_are_your_favorite_typescript_features_or/
- https://www.reddit.com/r/typescript/comments/1et3zhd/creating_a_library_best_practices/
- https://www.reddit.com/r/typescript/comments/151341h/typescriptreact_style_guide/
- https://www.reddit.com/r/reactjs/comments/vx9zir/has_typescript_made_you_a_better_developer/
- https://www.reddit.com/r/reactjs/comments/1hb663q/best_practices_for_integrating_typescript_into_a/
- https://www.reddit.com/r/typescript/comments/beiapy/enforce_your_team_coding_style_with_prettier_and/
- https://www.reddit.com/r/typescript/comments/18kj6tz/advice_on_getting_better_with_types/
- https://www.reddit.com/r/react/comments/1dsbpxp/seeking_large_react_typescript_projects_to_learn/
- https://www.reddit.com/r/vuejs/comments/1gdfnlq/typescript_style_guide/
- https://www.typescriptlang.org/docs/handbook/declaration-files/do-s-and-don-ts.html
- https://gist.github.com/anichitiandreea/e1d466022d772ea22db56399a7af576b
- https://dev.to/yugjadvani/how-to-write-better-typescript-code-best-practices-for-clean-effective-and-scalable-code-38d2
- https://docs.aws.amazon.com/prescriptive-guidance/latest/best-practices-cdk-typescript-iac/typescript-best-practices.html
- https://github.com/microsoft/TypeScript/wiki/Coding-guidelines
- https://dev.to/zenstack/11-tips-that-help-you-become-a-better-typescript-programmer-4ca1
- https://dev.to/stephengade/typescript-best-practices-part-1-55d5
- https://success.vanillaforums.com/kb/articles/226-coding-standard-typescript
- https://developer.mescius.com/blogs/typescript-tips-and-tricks
- https://engineering.zalando.com/posts/2019/02/typescript-best-practices.html
- https://www.telerik.com/blogs/mastering-typescript-benefits-best-practices
- https://softwaremill.com/4-typescript-tips-to-improve-your-developer-experience-that-you-might-not-know/
- https://google.github.io/styleguide/tsguide.html
- https://www.semanticscholar.org/paper/a36552b1b24db20b6879478dff01af4e77a77aa4
- https://www.semanticscholar.org/paper/8f0166b7fd750754be17601357b3bf49dcd0ebfd
- https://www.semanticscholar.org/paper/7dabc544c0fa2aecc08dd02eb1b12eb11231e23b
- https://www.semanticscholar.org/paper/bca46ee954f595b49c50b516d37b01432e4b167e
- https://www.reddit.com/r/typescript/comments/muyl55/understanding_args_never_in_typescript_handbook/
- https://www.reddit.com/r/typescript/comments/z8ygay/typescript_in_frontend/
- https://www.reddit.com/r/typescript/comments/1ezgsc9/announcing_typescript_56_rc/
- https://www.reddit.com/r/typescript/comments/fl59bk/important_rules_for_writing_idiomatic_typescript/
- https://www.reddit.com/r/typescript/comments/lpw9tu/what_are_your_problems_or_issues_with_developing/
- https://www.reddit.com/r/typescript/comments/13k1rlm/book_about_typescript/
- https://www.reddit.com/r/learnjavascript/comments/u7j266/why_dont_we_do_this_instead_of_typescript/
- https://www.reddit.com/r/javascript/comments/d7ryel/faq_private_vs_in_typescript/
- https://www.reddit.com/r/typescript/comments/1867eqi/is_typescript_a_genuinely_good_language_or_is_it/
- https://www.reddit.com/r/javascript/comments/rey2pu/askjs_how_heavy_do_you_lean_into_typescript/
- https://www.reddit.com/r/javascript/comments/d7ryel/faq_private_vs_in_typescript/?tl=pt-br
- https://www.reddit.com/r/typescript/comments/in51pa/does_typescript_check_types_at_runtime/
- https://www.reddit.com/r/typescript/comments/1hhtn96/typescript_interface_vs_type_differences_and_best/
- https://www.reddit.com/r/learnjavascript/comments/imoh86/what_are_the_pros_and_cons_of_using_typescript/
- https://blog.stackademic.com/mastering-typescript-conclusion-and-future-outlook-15a81681fb63
- https://www.typescriptlang.org
- https://velog.io/@seobbang/TypeScript-%ED%83%80%EC%9E%85%EC%8A%A4%ED%81%AC%EB%A6%BD%ED%8A%B8%EC%9D%98-%ED%83%80%EC%9E%85-%EC%8B%9C%EC%8A%A4%ED%85%9C
- https://www.typescriptlang.org/ko/docs/handbook/declaration-files/do-s-and-don-ts.html
- https://blog.stackademic.com/a-brief-summary-of-the-main-features-of-typescript-7fd97d576297
- https://velog.io/@yuyoni/SECTION-2.-%ED%83%80%EC%9E%85%EC%8A%A4%ED%81%AC%EB%A6%BD%ED%8A%B8-%EA%B8%B0%EC%B4%88-%ED%83%80%EC%9E%85-%EC%B6%94%EB%A1%A0-%ED%83%80%EC%9E%85-%EB%8B%A8%EC%96%B8-%ED%83%80%EC%9E%85-%EC%A2%81%ED%9E%88%EA%B8%B0
- https://news.hada.io/topic?id=19944
- https://www.typescriptlang.org/docs/handbook/typescript-in-5-minutes.html
- https://stackoverflow.com/questions/34754727/whats-the-point-of-this-section-of-code-in-typescript
- https://github.com/microsoft/typescript-go/discussions/categories/faqs
- https://www.heropy.dev/p/WhqSC8
- https://code.tutsplus.com/typescript-fundamentals–CRS-200881c/conclusion
- https://nomadcoders.co/faq/challenge/schedule-typescript
- https://www.semanticscholar.org/paper/a67eaa5b127d26645c03fa5e680a8efe3bd65007
- https://www.semanticscholar.org/paper/5cc7b69db7a891494eaf73e81401266ee5084cc2
- https://www.semanticscholar.org/paper/df797f880bd77bc6510cdc519216c712a85f36de
- https://www.semanticscholar.org/paper/0c572c7a50b55b924657f5ce0851accca1ba124e
- https://www.semanticscholar.org/paper/1551ebe29fab9b00d0a655c193f257e91e308a09
- https://www.semanticscholar.org/paper/b6b8b09789d033214217af5f6f403d6722ca3c0b
- https://www.reddit.com/r/typescript/comments/gpc10u/change_property_with_decorator_in_typescript/
- https://www.reddit.com/r/typescript/comments/11of3e4/any_benefit_to_migrating_to_new_decorators_from/
- https://www.reddit.com/r/typescript/comments/1alrphw/decorators/
- https://www.reddit.com/r/typescript/comments/m68mlh/can_anyone_help_me_understand_decorators/
- https://www.reddit.com/r/typescript/comments/worf22/has_anyone_successfully_created_a_dependency/
- https://github.com/arolson101/typescript-decorators
- https://www.social.plus/tutorials/using-decorators-in-typescript
- https://refine.dev/blog/typescript-decorators/
- https://reliasoftware.com/blog/decorators-in-typescript
- https://dev.to/baliachbryan/typescript-stage-3-decorators-a-journey-through-setup-and-usage-5f00
- https://www.semanticscholar.org/paper/26f70789edb9b0bce313f4c45c4b043a2f892f37
- https://arxiv.org/abs/2407.09453
- https://www.semanticscholar.org/paper/1f47bfec90ac7c5d1e796410b607d97157f746e8
- https://www.semanticscholar.org/paper/adde72d399a0d49cb992c080eb3a189680bf1814
- https://www.semanticscholar.org/paper/e79e715f9f9532d5b37af30f7620b95319f1354b
- https://www.reddit.com/r/AskProgramming/comments/ob7z80/is_typescript_a_compiled_language/
- https://www.reddit.com/r/typescript/comments/104ya7y/why_is_the_result_of_ts_to_js_so_special/
- https://www.reddit.com/r/typescript/comments/1i90zwp/how_do_i_compile_typescript_with_all_of_the/
- https://www.reddit.com/r/typescript/comments/1f3gnbo/why_does_my_typescript_transpilation_still_change/
- https://www.reddit.com/r/typescript/comments/1cdy0fj/output_all_typescript_files_to_a_javascript_file/
- https://www.reddit.com/r/typescript/comments/o8fzph/how_can_i_compile_the_typescript_project_while/
- https://nodejs.org/en/learn/typescript/transpile
- https://www.tutorialspoint.com/how-typescript-compilation-works
- https://huy.rocks/toylisp/04-01-2022-typescript-how-the-compiler-compiles
- https://www.youtube.com/watch?v=Pvg3B7jO6OU
- https://www.youtube.com/watch?v=X8k_4tZ16qU
- https://www.semanticscholar.org/paper/9e58b59c74ad12b10cd5ba767149aeea30ddb8a1
- https://pubmed.ncbi.nlm.nih.gov/29902338/
- https://www.semanticscholar.org/paper/87d4b683c98ecaee704bd426ce4791bc5c55ee5d
- https://www.semanticscholar.org/paper/6fdbfc16bd34abe2ecf9c9e7dcb171e3a54c8636
- https://www.semanticscholar.org/paper/a7b8f664d35d630fcc9a7afbb2a5d64e3944b10d
- https://www.reddit.com/r/typescript/comments/1f0yjrn/how_to_write_typescript_for_a_react_component/
- https://www.reddit.com/r/reactjs/comments/152zs2q/typescript_react_best_way_to_define_component/
- https://www.reddit.com/r/reactjs/comments/a4dc5m/is_it_worth_using_typescript_on_a_react_project/
- https://www.reddit.com/r/typescript/comments/l8omv5/looking_for_an_example_of_a_well_documented_react/
- https://www.reddit.com/r/typescript/comments/ih013n/learning_typescript_with_react_components/
- https://www.reddit.com/r/react/comments/10foj91/best_reactjs_practice_with/
- https://blog.logrocket.com/how-to-use-typescript-react-tutorial-examples/
- https://www.robinwieruch.de/typescript-react-component/
- https://www.electronforge.io/guides/framework-integration/react-with-typescript
- https://oida.dev/typescript-react/components/
- https://pubmed.ncbi.nlm.nih.gov/37199919/
- https://www.semanticscholar.org/paper/0c92d5e8d9811928e0fdb7fef8794b8bd881e74c